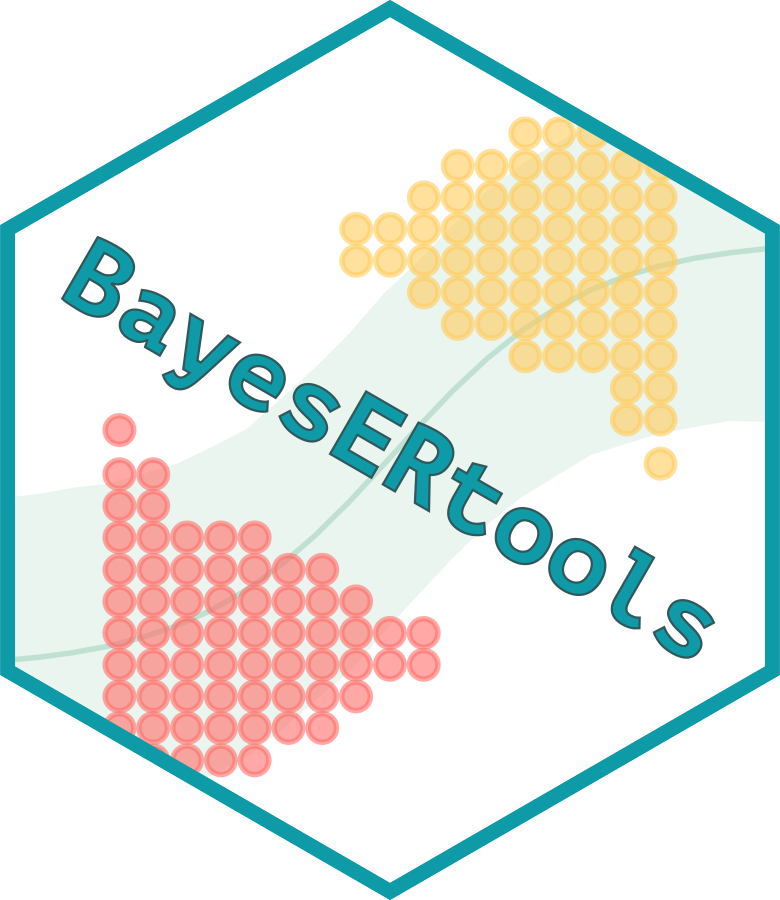
Exposure metrics selection for Emax models
dev_ermod_emax_exp_sel.Rd
This functions is used to develop an Emax model with binary and continuous endpoint, using various exposure metrics and selecting the best one.
Usage
dev_ermod_emax_exp_sel(
data,
var_resp,
var_exp_candidates,
verbosity_level = 1,
chains = 4,
iter = 2000,
gamma_fix = 1,
e0_fix = NULL,
emax_fix = NULL,
priors = NULL,
seed = sample.int(.Machine$integer.max, 1)
)
dev_ermod_bin_emax_exp_sel(
data,
var_resp,
var_exp_candidates,
verbosity_level = 1,
chains = 4,
iter = 2000,
gamma_fix = 1,
e0_fix = NULL,
emax_fix = NULL,
priors = NULL,
seed = sample.int(.Machine$integer.max, 1)
)
Arguments
- data
Input data for E-R analysis
- var_resp
Response variable name in character
- var_exp_candidates
Candidate exposure variable names in character vector
- verbosity_level
Verbosity level. 0: No output, 1: Display steps, 2: Display progress in each step, 3: Display MCMC sampling.
- chains
Number of chains for Stan.
- iter
Number of iterations for Stan.
- gamma_fix
Hill coefficient, default fixed to 1. See details in
rstanemax::stan_emax()
orrstanemax::stan_emax_binary()
- e0_fix
See details in
rstanemax::stan_emax()
orrstanemax::stan_emax_binary()
- emax_fix
See details in
rstanemax::stan_emax()
orrstanemax::stan_emax_binary()
- priors
See details in
rstanemax::stan_emax()
orrstanemax::stan_emax_binary()
- seed
Random seed for Stan model execution, see details in
rstan::sampling()
which is used inrstanemax::stan_emax()
orrstanemax::stan_emax_binary()
Examples
# \donttest{
data_er_cont <- rstanemax::exposure.response.sample
noise <- 1 + 0.5 * stats::rnorm(length(data_er_cont$exposure))
data_er_cont$exposure2 <- data_er_cont$exposure * noise
# Replace exposure < 0 with 0
data_er_cont$exposure2[data_er_cont$exposure2 < 0] <- 0
ermod_emax_exp_sel <-
dev_ermod_emax_exp_sel(
data = data_er_cont,
var_resp = "response",
var_exp_candidates = c("exposure", "exposure2")
)
#> ℹ The exposure metric selected was: exposure
ermod_emax_exp_sel
#>
#> ── Emax model & exposure metric selection ──────────────────────────────────────
#> ℹ Use `plot_er_exp_sel()` for ER curve of all exposure metrics
#> ℹ Use `plot_er()` with `show_orig_data = TRUE` for ER curve of the selected exposure metric
#>
#> ── Exposure metrics comparison ──
#>
#> elpd_diff se_diff
#> exposure 0.00 0.00
#> exposure2 -9.46 3.30
#>
#> ── Selected model ──
#>
#> ---- Emax model fit with rstanemax ----
#> mean se_mean sd 2.5% 25% 50% 75% 97.5% n_eff Rhat
#> emax 92.01 0.12 6.07 80.13 88.01 92.02 96.20 103.86 2418.78 1
#> e0 5.72 0.10 4.72 -3.63 2.54 5.74 8.88 14.96 2085.81 1
#> ec50 75.96 0.49 20.45 45.07 61.24 73.36 87.46 124.72 1769.73 1
#> gamma 1.00 NaN 0.00 1.00 1.00 1.00 1.00 1.00 NaN NaN
#> sigma 16.63 0.03 1.56 13.92 15.50 16.51 17.58 19.96 2735.09 1
#> * Use `extract_stanfit()` function to extract raw stanfit object
#> * Use `extract_param()` function to extract posterior draws of key parameters
#> * Use `plot()` function to visualize model fit
#> * Use `posterior_predict()` or `posterior_predict_quantile()` function to get
#> raw predictions or make predictions on new data
#> * Use `extract_obs_mod_frame()` function to extract raw data
#> in a processed format (useful for plotting)
# }
# \donttest{
data_er_bin <- rstanemax::exposure.response.sample.binary
noise <- 1 + 0.5 * stats::rnorm(length(data_er_bin$conc))
data_er_bin$conc2 <- data_er_bin$conc * noise
data_er_bin$conc2[data_er_bin$conc2 < 0] <- 0
ermod_bin_emax_exp_sel <-
dev_ermod_bin_emax_exp_sel(
data = data_er_bin,
var_resp = "y",
var_exp_candidates = c("conc", "conc2")
)
#> ℹ The exposure metric selected was: conc
# }