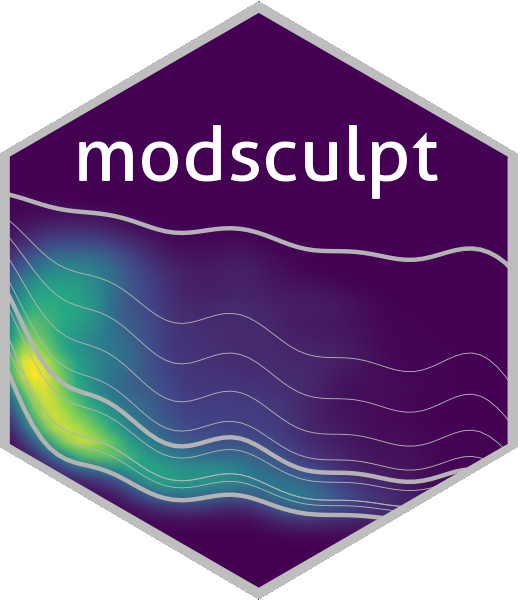
Create a rough model
sculpt_rough.Rd
Create a rough model
Usage
sculpt_rough(
dat,
model_predict_fun,
n_ice = 10,
seed = NULL,
verbose = 0,
allow_par = FALSE,
model_predict_fun_export = NULL,
data_as_marginals = FALSE
)
Arguments
- dat
Data to create the rough model from. Must be a product marginal dataset (see
sample_marginals
) with covariates only (i.e. without response).- model_predict_fun
Function that returns predictions given a dataset.
- n_ice
Number of ICE curves to generate. Defaults to 10.
- seed
(
NULL
) or seed for exact reproducibility.- verbose
(
integer
) 0 for silent run, > 0 for messages.- allow_par
(
logical
) Allow parallel computation? Defaults toFALSE
.- model_predict_fun_export
For parallel computation only. If there is a parallel backend registered (see
parallel_set()
), then use this to export variables used inmodel_predict_fun
(like model). This is passed toforeach::foreach(..., .export = model_predict_fun_export)
.- data_as_marginals
(
logical
) Use the provided datadat
as already sampled dataset? Defaults toFALSE
.
Details
For parallel computation, use parallel_set()
and set allow_par
to TRUE
.
Note that parallel computation may fail if the model is too big and there is not enough memory.
Examples
df <- mtcars
df$vs <- as.factor(df$vs)
model <- rpart::rpart(
hp ~ mpg + carb + vs,
data = df,
control = rpart::rpart.control(minsplit = 10)
)
model_predict <- function(x) predict(model, newdata = x)
covariates <- c("mpg", "carb", "vs")
pm <- sample_marginals(df[covariates], n = 50, seed = 5)
rs <- sculpt_rough(
dat = pm,
model_predict_fun = model_predict,
n_ice = 10,
seed = 1,
verbose = 0
)
class(rs)
#> [1] "rough" "sculpture" "list"
head(predict(rs))
#> 1 2 3 4 5 6
#> 94.25971 177.04543 136.83114 174.91686 136.83114 136.83114
# lm model without interaction -> additive -> same predictions
model <- lm(hp ~ mpg + carb + vs, data = df)
model_predict <- function(x) predict(model, newdata = x)
covariates <- c("mpg", "carb", "vs")
pm <- sample_marginals(df[covariates], n = 50, seed = 5)
rs <- sculpt_rough(
dat = pm,
model_predict_fun = model_predict,
n_ice = 10,
seed = 1,
verbose = 0
)
class(rs)
#> [1] "rough" "sculpture" "list"
head(predict(rs))
#> 1 2 3 4 5 6
#> 94.61787 190.78181 150.66447 143.70887 126.25460 102.95408
head(predict(model, pm))
#> 1 2 3 4 5 6
#> 94.61787 190.78181 150.66447 143.70887 126.25460 102.95408